Python Lists Guide: Everything You Need to Know
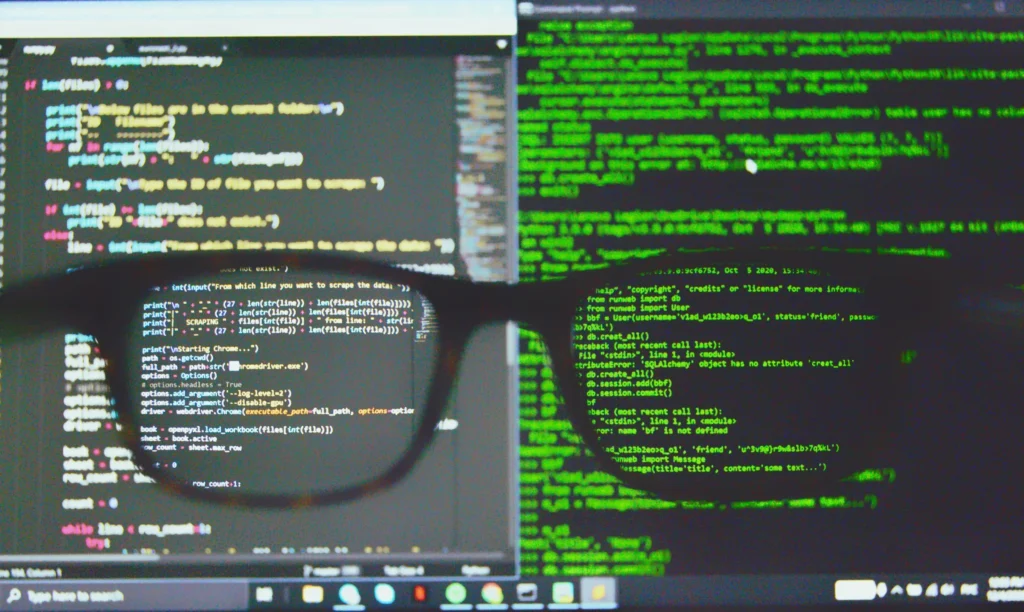
In the vast realm of Python programming, lists stand as one of the fundamental data structures that every developer should grasp. Whether you’re a seasoned Python programmer or just starting out, understanding Python lists is crucial for building efficient and effective applications. In this comprehensive guide, we will delve deep into Python lists, exploring their features, functions, and best practices.
By the end of this article, you’ll have a solid grasp of Python lists and be well-equipped to harness their power in your coding endeavors.
What Are Python Lists?
In Python, a list is a versatile and ordered collection of items. These items can be of any data type – integers, strings, floats, or even other lists. Lists are denoted by square brackets `[ ]` and are separated by commas. Lists can dynamically grow or shrink, making them flexible containers for various data.
Creating Lists
To create a list, simply enclose a series of items within square brackets and separate them with commas. Here’s an example:
my_list = [1, 2, 3, 'apple', 'banana', 3.14]
Lists can contain elements of different data types, as shown in the above example.
Retrieving List Elements
Accessing individual elements in a list is straightforward. Lists are zero-indexed, meaning the first element is at index 0, the second at 1, and so on. To access an element, use square brackets with the index:
fruits = ['apple', 'banana', 'cherry']
print(fruits[0]) # Output: 'apple'
Lists Appending and Extending
Lists are mutable, which means you can change their elements. Here are some common list modifications:
To add an element to the end of a list, you can use the `append()` method:
fruits = ['apple', 'banana']
fruits.append('cherry')
# Now, fruits contains ['apple', 'banana', 'cherry']
To extend a list with another list, use the `extend()` method:
fruits = ['apple', 'banana']
fruits.extend(['cherry', 'date'])
# fruits now contains ['apple', 'banana', 'cherry', 'date']
Inserting Elements
You can insert an element at a specific position using the `insert()` method:
fruits = ['apple', 'banana', 'date']
fruits.insert(1, 'cherry')
# fruits now contains ['apple', 'cherry', 'banana', 'date']
Removing Elements
To remove elements, you can use the `remove()` method:
fruits = ['apple', 'banana', 'cherry']
fruits.remove('banana')
# fruits now contains ['apple', 'cherry']
List Methods
Python lists come with a variety of built-in methods for manipulating data. Some commonly used methods include:
- append(): Adds an element to the end of the list.
- extend(): Appends the elements of another list to the end.
- insert(): Inserts an element at a specific position.
- remove(): Removes the first occurrence of a value.
- pop(): Removes and returns an element at a specified index.
- index(): Returns the index of the first occurrence of a value.
- count(): Returns the number of times a value appears in the list.
- sort(): Sorts the list in ascending order.
- reverse(): Reverses the order of elements.
List Comprehensions
List comprehensions are a concise way to create lists in Python. They provide a compact and readable syntax for generating lists based on existing lists.
Here’s an example:
squares = [x ** 2 for x in range(1, 6)]
# squares will be [1, 4, 9, 16, 25]
Working with Nested Lists
Python allows you to create lists of lists, often referred to as nested lists. These can represent multi-dimensional data structures, such as matrices. Accessing elements in nested lists requires multiple indices. For instance:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(matrix[1][2]) # Output: 6
Slicing and Dicing Lists
Slicing allows you to extract a portion of a list. You specify a range of indices to create a new list that includes the elements within that range. For example:
numbers = [0, 1, 2, 3, 4, 5]
subset = numbers[2:4]
# subset will be [2, 3]
List Operations and Functions
In addition to methods, you can perform various operations on lists, including concatenation and repetition:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Concatenation
result = list1 + list2 # [1, 2, 3, 4, 5, 6]
# Repetition
repeated = list1 * 3 # [1, 2, 3, 1, 2, 3, 1, 2, 3]
Common Pitfalls
When working with Python lists, it’s important to be aware of potential pitfalls. One common issue is “aliasing,” where multiple variables reference the same list, leading to unintended changes when modifying it. Another pitfall is the difference between shallow and deep copies.
Shallow copies create a new list but still reference the original objects, while deep copies create entirely independent copies. Be cautious when dealing with these to avoid unexpected results and side effects in your code.
Best Practices for Python Lists
To write efficient and maintainable Python code, consider these best practices when working with lists. Choose the right data structures for your needs, as different data structures can offer better performance for specific tasks.
List comprehensions are a concise way to create and manipulate lists, so use them when appropriate to improve readability. Additionally, optimize loops for better performance by avoiding unnecessary iterations and using built-in functions.
Use Cases and Examples
Python lists have versatile applications, such as data processing, creating collections of information, and managing data.
For instance, you can use lists to store a list of names, keep track of scores, or process data from a file. Let’s explore real-world examples to illustrate how lists can be valuable in these scenarios.
Advanced Topics
For advanced users, there are more powerful techniques to master. Dive into list comprehensions to create and transform lists efficiently. Learn about advanced list manipulation methods, like sorting, filtering, and slicing, to streamline your code.
Discover complex use cases where lists shine, such as implementing data structures like stacks or queues.
Performance Considerations
Efficiency is crucial in programming. Understand the performance considerations of lists, including time complexity (how long an operation takes) and memory usage (how much memory is consumed).
Efficiently selecting and manipulating data in lists can make your code faster and more memory-efficient.
Conclusion
Python lists are indispensable tools in a programmer’s toolkit. Their flexibility and versatility make them suitable for a wide range of tasks.
By avoiding common pitfalls, following best practices, and exploring advanced techniques, you can harness the full potential of Python lists in your coding adventures.
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends