30 Essential Django Interview Questions
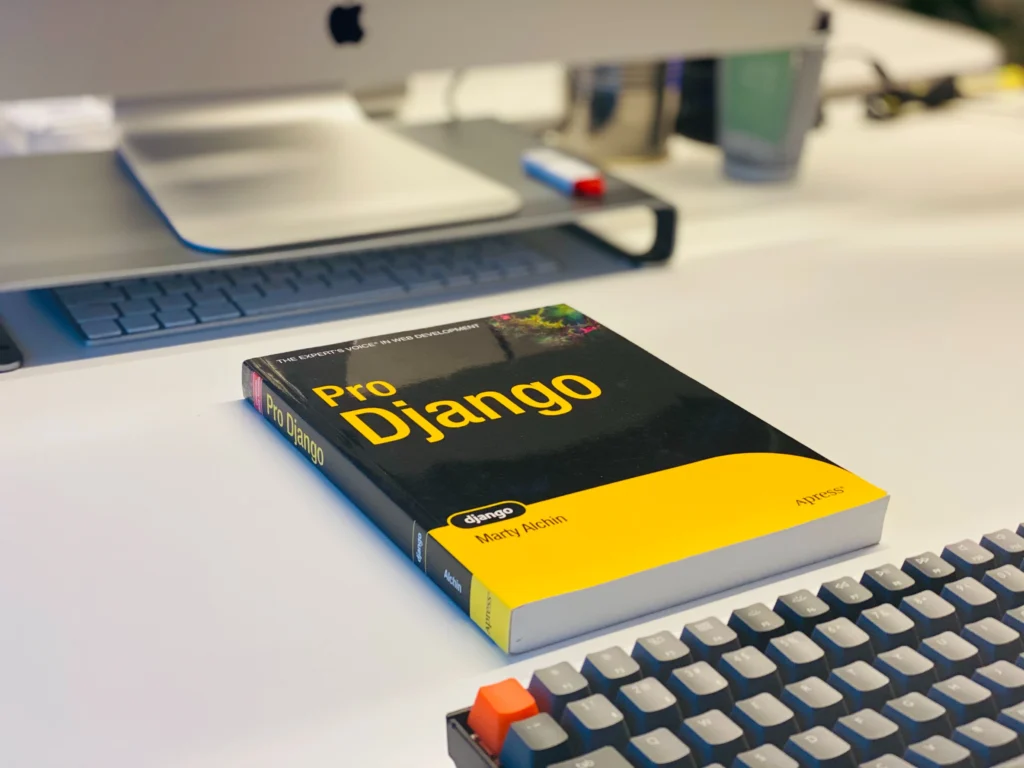
We’ll explore fundamental concepts, core features, and some advanced aspects of Django while keeping the explanations simple and practical.
Django is one of the most popular web frameworks for developing Python applications. Known for its simplicity, flexibility, and rapid development capability, Django has a strong following among developers and businesses. Whether you’re a job-seeker preparing for an interview or a hiring manager putting together interview questions, this article covers 30 essential Django interview questions to get you started.
1. What is Django?
Django is an open-source web framework written in Python. It follows the Model-View-Template (MVT) architectural pattern, which separates the application’s business logic from its presentation layer. Django aims to make web development easier and faster by providing built-in tools for database handling, URL routing, form handling, and more.
2. What are Django’s main features?
Key features of Django include:
- Fast Development: Helps developers create applications quickly by handling repetitive tasks.
- Scalability: Designed to handle high-traffic sites.
- Security: Provides security features to protect against attacks such as SQL injection, XSS, and CSRF.
- Versatile Database Management: Works well with multiple databases and provides Object-Relational Mapping (ORM).
3. Explain the MVT architecture in Django.
In Django, MVT stands for Model-View-Template:
- Model: Represents the database schema and handles data.
- View: Contains the business logic and processes user requests.
- Template: Manages how the data is presented to the user.
Django’s MVT pattern helps keep the application structured and modular.
4. What is Django ORM, and why is it useful?
Django’s Object-Relational Mapper (ORM) is a tool that lets developers interact with databases using Python code instead of SQL. With Django ORM, you can create and query databases without writing raw SQL queries. This improves code readability, and portability, and reduces errors.
5. How does Django handle database migrations?
Django uses a tool called migrations to manage changes in the database schema. When you make changes to models, you can run python manage.py makemigrations to generate migration files. Then, use python manage.py migrate to apply these migrations to the database.
6. What is a Django model?
A Django model is a Python class that represents a table in a database. Each model defines the fields and behaviors of the data you’re working with. Models are used to create, retrieve, update, and delete records in the database.
Recommended Article: OOP in Python
7. Explain the role of Django’s urls.py file.
The urls.py file is responsible for mapping URLs to views. Django’s URL dispatcher routes incoming requests to the appropriate view based on patterns defined in urls.py. This enables Django to match requested URLs with specific actions within the application.
8. What is a Django view?
A Django view is a function or class that handles a request and returns a response. Views contain the logic for what to do with a user’s request. There are two types of views:
- Function-based views: Python functions that take a request and return a response.
- Class-based views: Offer more functionality and are ideal for views with repetitive code.
9. What are Django templates?
Django templates define how data will be displayed to users. They use the Django Template Language (DTL) to render HTML with dynamic data. Templates make it easy to separate presentation from business logic, ensuring a clean codebase.
10. How do you use static files in Django?
Django manages static files like CSS, JavaScript, and images using the STATIC_URL and STATICFILES_DIRS settings. In production, Django collects all static files into a single directory using the collectstatic command, making it easier to serve them efficiently.
11. What is middleware in Django?
Middleware is a set of components that process requests and responses. It sits between the server and the view, modifying requests or responses as needed. Common examples include security middleware for handling headers, session middleware for user sessions, and authentication middleware.
See the Detailed Guide on middleware in Django here.
12. What is Django’s settings.py file?
The settings.py file is the main configuration file for a Django project. It stores important settings like database configurations, installed apps, middleware, static files, and more. Customizing settings.py allows developers to control application behavior.
13. How do you create a Django project?
To create a Django project, run:
django-admin startproject projectname
This command sets up the initial project structure, including settings.py, urls.py, wsgi.py, and asgi.py.
14. How do you create a Django app?
Inside a Django project, apps are used to separate components of an application. To create an app, run:
bash
python manage.py startapp appname
This command creates a new app directory with files like models.py, views.py, tests.py, and apps.py.
15. What is Django’s manage.py file?
manage.py is a command-line utility for managing Django projects. It allows you to run commands like starting the development server, creating migrations, managing databases, and running tests.
16. Explain Django’s @login_required decorator.
The @login_required decorator restricts access to views so that only authenticated users can access them. It redirects unauthenticated users to a login page and is commonly used in applications that require user authentication.
17. What is Django’s get_object_or_404 function?
get_object_or_404 is a Django shortcut that retrieves an object from the database or returns a 404 error if the object doesn’t exist. This helps avoid errors and improves user experience by showing an error page for non-existent resources.
18. What is Django Admin, and how do you use it?
Django Admin is a built-in application for managing data models. It provides a user-friendly interface to view, add, edit, and delete records. To use Django Admin, register your models in admin.py, and you can then access the admin dashboard by visiting /admin in your application.
You may like: How to send Email in Python.
19. How does Django handle form validation?
Django provides form classes that allow developers to validate user input. By using the is_valid() method, Django checks each form field against the defined validators and raises errors if the input doesn’t meet the criteria. This makes data validation simple and secure.
20. What is Django’s ForeignKey?
ForeignKey is a Django field used to define relationships between models, specifically a many-to-one relationship. For example, a blog post model might have a ForeignKey linking it to an author model, indicating that each post is written by one author.
21. How do you filter queries in Django?
Django’s ORM provides the filter() method to query data based on specific criteria. For instance, to retrieve all posts by a particular author, you might use:
posts = Post.objects.filter(author=author)
22. What is the purpose of __str__ method in Django models?
The __str__ method in Django models returns a human-readable representation of an object. It’s used in the Django Admin and other places to display objects as strings. For example:
def __str__(self):
return self.title
23. Explain Django signals.
Signals in Django allow decoupled applications to get notified when specific actions occur. Common signals include post_save, which triggers after an object is saved, and pre_delete, which triggers before an object is deleted. Signals can be useful for actions like updating related records or sending notifications.
24. What is the purpose of Django’s Meta class in models?
The Meta class in Django models allows you to configure certain behaviors of the model, such as ordering, verbose names, unique constraints, and database table names. For example, to order blog posts by their creation date in descending order, you would use:
class Post(models.Model):
title = models.CharField(max_length=100)
created_at = models.DateTimeField(auto_now_add=True)
class Meta:
ordering = ['-created_at']
25. What are Django’s OneToOneField, ForeignKey, and ManyToManyField?
Django provides three types of fields for establishing relationships between models:
- OneToOneField: Creates a one-to-one relationship between two models. This is often used for profiles, where each user has one profile.
- ForeignKey: Represents a many-to-one relationship. For example, a blog post may have a foreign key to an author, meaning one author can have many posts.
- ManyToManyField: Defines a many-to-many relationship. For instance, a blog post can have multiple tags, and a tag can belong to multiple posts.
26. What are Django Model Managers?
Model Managers in Django are used to manage the querying of models. The default manager is objects, but custom managers can be created by subclassing models. Manager. Custom managers allow you to define methods that encapsulate complex query logic, making it easier to reuse that logic across the application.
For example, a custom manager to get published posts might look like this:
class PublishedManager(models.Manager):
def get_queryset(self):
return super().get_queryset().filter(status='published')
27. Explain the difference between null=True and blank=True in Django models.
In Django models:
- null=True: Allows database fields to store NULL (empty) values.
- blank=True: Controls whether a field is required when using forms. If set to True, the field is optional in forms.
For example, a description field in a model might have null=True, and blank=True if it’s optional both in the database and form.
28. How does Django handle security for forms?
Django provides several built-in mechanisms to protect against security threats in forms:
- Cross-Site Request Forgery (CSRF): Django includes a CSRF token in forms to prevent CSRF attacks.
- Cross-Site Scripting (XSS): Django automatically escapes variables in templates to protect against XSS.
- SQL Injection: The Django ORM escapes SQL queries to prevent SQL injection attacks.
- Clickjacking Protection: Django provides X-Frame-Options headers by default to prevent clickjacking.
29. What is caching in Django, and how is it implemented?
Caching in Django improves performance by storing the results of expensive computations or frequently accessed data. Django offers various caching strategies, such as in-memory caching, file-based caching, and using external caching services like Memcached or Redis.
To enable caching, configure a cache in settings.py and use Django’s caching tools like cache.set and cache.get to manage cached data.
30. How can you deploy a Django application?
Deploying a Django application involves several steps:
- Choose a Server: Deploy the application on a server, such as DigitalOcean, AWS, or Heroku.
- Configure the Web Server: Use web servers like Nginx or Apache to serve the application.
- Use Gunicorn or uWSGI: These WSGI servers interface between the web server and Django.
- Set up the Database: Configure your production database, often PostgreSQL or MySQL.
- Serve Static and Media Files: Use a content delivery network (CDN) or configure your server to serve these files.
- Environment Variables: Use .env files or environment variables for sensitive data like API keys and secret keys.
- Configure Debug Mode: Set DEBUG = False for production to prevent exposing sensitive information.
Tips for Preparing Django Interview Questions
As a job candidate, thoroughly understanding these 30 Django interview questions will prepare you well for an interview. Here are a few additional tips to help you excel:
- Practice Hands-On: Reading about Django is great, but hands-on experience is essential. Try creating small projects or extending existing ones to become more familiar with concepts like models, views, forms, and Django ORM.
- Build an End-to-End Project: Having a real project in your portfolio can help demonstrate your skills. A simple blog application, e-commerce site, or social media platform clone can showcase your understanding of Django’s core features.
- Explore Advanced Topics: Once you’re comfortable with the basics, dive into advanced concepts like Django REST Framework (DRF), custom middleware, and caching techniques. Many companies value candidates with experience in these areas.
- Understand Common Patterns: Familiarize yourself with Django’s common design patterns, like how to use decorators, handle form validation, and implement pagination. Knowing these patterns helps show that you can write clean, maintainable code.
- Stay Updated: Django is constantly evolving. Be aware of the latest version, its features, and its improvements. Reading Django’s official documentation or following community blogs can keep you up-to-date.
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends