Python Exception Handling
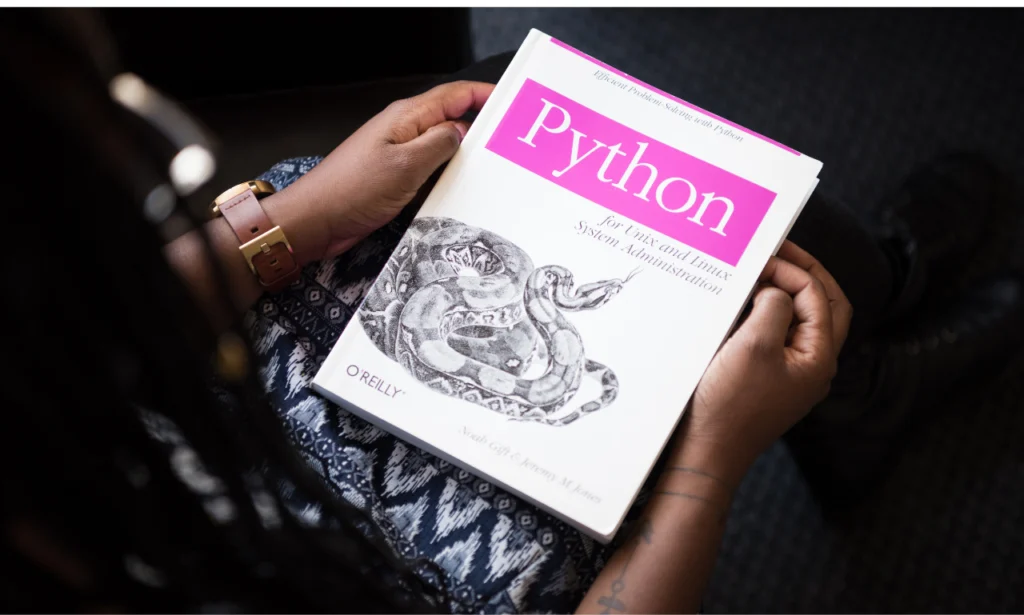
Exception handling in Python is like having a safety net for your code – it helps your program handle unexpected bumps without crashing. Let’s dive into the world of Python exception handling, exploring the `try`, `except`, `else`, and `finally` blocks that make your code more resilient.
The ‘try’ Block:
Imagine the `try` block as a protective bubble around the part of your code that might encounter issues. It’s the space where Python keeps an eye out for potential errors. Everything inside is executed step by step until either an exception occurs or the block finishes without any hiccups.
try:
# Code that might cause trouble
result = 10 / 0 # An example of a problematic operation (division by zero)
except Exception as e:
# What to do when things go wrong
print(f"Uh-oh! An issue popped up: {e}")
The ‘except’ Block:
When something goes awry inside the `try` block, the `except` block steps in. It’s like a superhero ready to tackle specific types of problems. You can have different `except` blocks for different issues, making your code handle errors in a tailored manner.
except ZeroDivisionError:
print("Oops! Dividing by zero is a no-go.")
except Exception as e:
print(f"Something unexpected happened: {e}")
The ‘else’ block is the silver lining – it only runs if everything in the `try` block goes smoothly. It’s optional but handy for keeping your error-handling logic separate from the regular code.
else:
print("Smooth sailing! No errors here.")
The ‘finally’ Block:
No matter what, the `finally` block always runs. It’s the cleanup crew, perfect for tasks like closing files or releasing resources. This block ensures your code tidies up, even if there were bumps along the way.
finally:
print("This part always runs, whether there were errors or not.")
Related: For loops in Python
Exception Hierarchy:
Think of Python’s exception hierarchy as a family tree, where specific issues are like the children inheriting traits from a more general parent. Knowing this hierarchy helps you target specific problems and handle them with finesse.
try:
# Code susceptible to issues
except SpecificException: # Dealing with a specific problem
#code
except GeneralException as e: # Handling a broader problem with extra info
#code
In a nutshell, Python exception handling is your code’s safety blanket. By using `try`, `except`, `else`, and `finally`, you create a program that gracefully handles errors, making it more robust and reliable. Dive into the exception hierarchy to fine-tune your responses to specific issues. It’s all about keeping your code not just functional, but resilient in the face of the unexpected.
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends