Unlock Web Scraping with Scrapy in Python: Techniques, Tools, and Best Practices
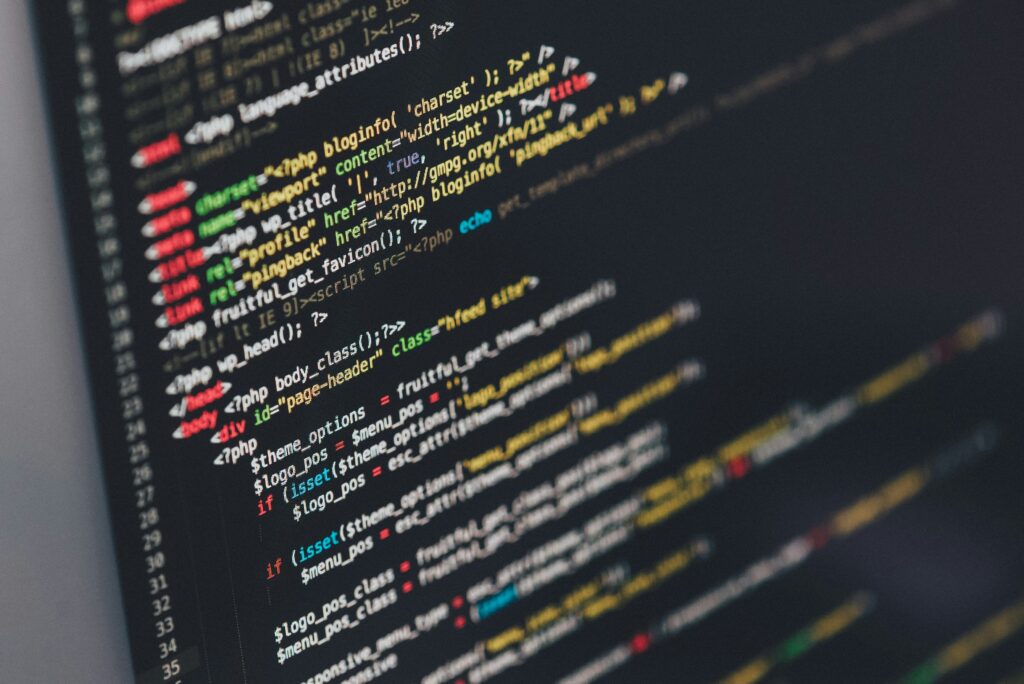
Web Scraping with Scrapy in Python
Introduction to Web Scraping and Scrapy
What is Web Scraping?
Web scraping is the process of extracting data from websites. It’s a powerful technique used for a wide range of purposes, from competitive price monitoring, lead generation, and market research to collecting data for machine learning models or automated workflows. Web scraping allows you to gather data from multiple websites and aggregate it into a structured format (like CSV, JSON, or databases), helping businesses make data-driven decisions more efficiently.
For example, if you’re tracking product prices across e-commerce websites or gathering real estate listings for analysis, web scraping becomes invaluable. The manual task of visiting pages and collecting data can be automated, saving both time and effort.
However, web scraping comes with challenges such as dealing with dynamic content, CAPTCHA systems, and rate-limiting mechanisms that are designed to prevent bots. Hence, having a robust framework like Scrapy can make web scraping much easier and more scalable.
Overview of Scrapy Framework
Scrapy is a popular open-source Python framework for web scraping. It allows developers to easily write spiders (custom web crawlers) that navigate web pages and extract the required data in a structured manner. Web scraping with Scrapy is more powerful than traditional scraping libraries like BeautifulSoup because it includes built-in support for:
- Handling requests and responses.
- Extracting data using CSS selectors or XPath.
- Managing multiple concurrent requests efficiently.
- Automatically following links (crawling).
Storing scraped data in various formats, including JSON, CSV, and databases.
What makes Scrapy stand out is its ability to handle large-scale scraping projects. With Scrapy, you can build spiders that not only scrape individual pages but also traverse across multiple pages, making it a powerful tool for crawling entire websites.
For larger projects, Scrapy offers advanced features like integrating middleware, pipelines, and support for deploying spiders on cloud services like Scrapy Cloud or AWS.
Setting Up Scrapy
Prerequisites for Scrapy
Before getting started with Scrapy, you need some basic prerequisites:
- Python Environment: Scrapy requires Python to be installed on your system. You can download the latest version of Python from the [official website].
- Pip (Python Package Installer): Scrapy is installed via pip, so make sure you have it set up by default with Python. You can check by running:
pip --version
Installation of Scrapy
Once you have Python and pip ready, installing Scrapy is simple. Open your terminal and run the following command:
pip install scrapy
This will install Scrapy and all its dependencies, allowing you to start building your scraping projects.
Creating Your First Scrapy Project
To create a new Scrapy project, navigate to the directory where you want to store your project and run:
scrapy startproject myproject
This command will generate the basic directory structure of a Scrapy project:
myproject/
scrapy.cfg
myproject/
__init__.py
items.py
middlewares.py
pipelines.py
settings.py
spiders/
__init__.py
Here’s a brief overview of the key files:
- scrapy.cfg: Configuration file for the entire project.
- items.py: Define the data structure of the items you’re scraping.
- spiders/: This folder contains your spiders—these are the scripts responsible for fetching the data.
- settings.py: A place to configure your project’s settings like user-agent, concurrent requests, and download delays.
Once your project is set up, you can begin writing your first spider.
Building Your First Spider
Understanding Spiders in Scrapy
A spider in Scrapy is a class that defines how to follow links on a website and extract the necessary information. Each spider contains a few essential components:
- start_requests(): Defines the initial URLs the spider should begin scraping from.
- parse(): A callback method that processes the response from a website and extracts data.
- Custom callback functions: If needed, you can define additional functions to handle special scraping cases.
Writing Your First Spider
Let’s create a simple spider that scrapes quotes from a sample website. Start by creating a new file inside the spiders/ directory, named quotes_spider.py, with the following code:
import scrapy
class QuotesSpider(scrapy.Spider):
name = "quotes"
start_urls = ['http://quotes.toscrape.com/']
def parse(self, response):
for quote in response.css('div.quote'):
yield {
'text': quote.css('span.text::text').get(),
'author': quote.css('small.author::text').get(),
'tags': quote.css('div.tags a.tag::text').getall(),
}
next_page = response.css('li.next a::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
Here’s what’s happening in this spider:
- name: The unique name of the spider, used to run it later.
- start_urls: The initial URL where the spider starts scraping.
- parse(): The method that extracts data. We’re using CSS selectors to target specific elements (like the quote text, author, and tags).
- next_page: The spider follows pagination links to scrape data from multiple pages.
To run this spider, navigate to the project’s root directory and type:
scrapy crawl quotes
This will start the spider, scrape the quotes, and print the results in the terminal. You can also export the scraped data into a JSON file:
scrapy crawl quotes -o quotes.json
This command will save all scraped data into quotes.json.
Recommended Content: How to send e-mail in Python
Advanced Scraping Techniques
As you start to deal with more complex websites, you’ll encounter challenges like dynamic content, pagination, and handling forms. In this section, we’ll dive into some advanced scraping techniques with Scrapy to tackle these common challenges.
Handling Dynamic Content with Scrapy
Many modern websites use JavaScript to dynamically load content, making it harder for Scrapy to scrape the data. Scrapy only retrieves the static HTML content, which may not include data generated by JavaScript.
To handle this, we have two main approaches:
Integrating Scrapy with Selenium
- Selenium is a Python library that automates web browsers and can be used to load JavaScript-driven content.
- You can use Selenium to render the dynamic page, and then pass the HTML content to Scrapy for extraction.
Example:
import scrapy
from selenium import webdriver
from scrapy import Selector
class MySpider(scrapy.Spider):
name = 'selenium_spider'
def start_requests(self):
driver = webdriver.Chrome() # Use appropriate webdriver (e.g., Chrome)
driver.get('http://example.com')
sel = Selector(text=driver.page_source)
# Extract your data using Scrapy's selectors
data = sel.css('div.some-class::text').getall()
driver.quit()
# Process the data here
yield {'data': data}
This is just an example template code, you can get more info from here.
Using Scrapy-Splash for JavaScript Rendering
- Another alternative is Scrapy-Splash, a headless browser that integrates with Scrapy and renders JavaScript content.
- You can set up Splash with Docker and use it to render dynamic pages within your Scrapy project.
To use Scrapy-Splash, install it with:
pip install scrapy-splash
Update your Scrapy settings to include the Splash middleware, and your spider to request dynamic pages like this:
import scrapy
from scrapy_splash import SplashRequest
class QuotesSpider(scrapy.Spider):
name = "js_spider"
start_urls = ['http://quotes.toscrape.com/js/']
def start_requests(self):
for url in self.start_urls:
yield SplashRequest(url, self.parse, args={'wait': 2})
def parse(self, response):
for quote in response.css('div.quote'):
yield {
'text': quote.css('span.text::text').get(),
'author': quote.css('small.author::text').get(),
'tags': quote.css('div.tags a.tag::text').getall(),
}
For more info on scrapy + scrapy-splash, refer here.
Dealing with Pagination
Many websites split content across multiple pages (pagination). Scrapy makes it easy to handle pagination with a few simple adjustments.
In the earlier example, we already handled pagination with the next_page logic. Here’s a more detailed look at how to handle pagination:
1. Locate the Next Page Link:
– Use CSS or XPath selectors to find the URL for the Next button or link on the page.
2. Follow the Pagination Links:
– Use Scrapy’s response.follow to automatically follow pagination links.
Here’s a complete example of a spider scraping articles from multiple pages:
import scrapy
class ArticleSpider(scrapy.Spider):
name = "articles"
start_urls = ['http://example.com/articles']
def parse(self, response):
for article in response.css('div.article'):
yield {
'title': article.css('h2::text').get(),
'author': article.css('span.author::text').get(),
'date': article.css('span.date::text').get(),
}
next_page = response.css('li.next a::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
This spider extracts data from each article and follows the pagination links until there are no more pages left.
Recommended article: RAG vs finetuning
Handling Forms and User Inputs
Sometimes, the data you want to scrape is hidden behind forms or requires specific user inputs (e.g., login forms or search filters). Scrapy supports making POST requests to submit forms and retrieve the relevant data.
Here’s how you can send a POST request in Scrapy:
1. Find the Form Data:
– Use browser developer tools to inspect the form and find the necessary fields (like CSRF tokens or session IDs).
2. Submit the Form with Scrapy:
import scrapy
class LoginSpider(scrapy.Spider):
name = 'login'
start_urls = ['http://example.com/login']
def parse(self, response):
return scrapy.FormRequest.from_response(
response,
formdata={'username': 'myuser', 'password': 'mypassword'},
callback=self.after_login
)
def after_login(self, response):
if "incorrect" in response.body.decode('utf-8'):
self.logger.error("Login failed")
return
# Proceed with scraping after login
return scrapy.Request(url='http://example.com/profile', callback=self.parse_profile)
def parse_profile(self, response):
yield {'profile_data': response.css('div.profile::text').get()}
This spider logs in to a website and then proceeds to scrape a protected profile page after a successful login.
Data Extraction and Storage
Scrapy Selectors and XPath
Scrapy offers powerful data extraction methods through CSS selectors and XPath. Understanding how to use these selectors efficiently is crucial to extracting specific data from a webpage.
1. CSS Selectors: Use these to target HTML elements by their tag names, class names, or IDs.
Example:
response.css('div.quote span.text::text').get()
– This selector extracts the text of the span element inside the div with class `quote`.
2. XPath Selectors: XPath is a query language for selecting nodes from an XML-like document structure.
Example:
response.xpath('//div[@class="quote"]/span[@class="text"]/text()').get()
– This XPath extracts the text content of the same element as the CSS example above.
Storing Scraped Data
Once you’ve scraped the data, you’ll need to store it for later use. Scrapy makes it easy to save data in various formats, including JSON, CSV, and databases like PostgreSQL or MongoDB.
1. Storing Data in JSON or CSV:
– To export your data, you can use Scrapy’s built-in command-line options:
scrapy crawl quotes -o quotes.json # JSON
scrapy crawl quotes -o quotes.csv # CSV
2. Using Scrapy Pipelines for Databases:
If you need to save data directly to a database, Scrapy provides pipelines. You define pipelines in the pipelines.py file and configure them in settings.py. Here’s a basic example of storing data in a MongoDB database:
import pymongo
class MongoPipeline:
def open_spider(self, spider):
self.client = pymongo.MongoClient("mongodb://localhost:27017/")
self.db = self.client["scrapy_db"]
def close_spider(self, spider):
self.client.close()
def process_item(self, item, spider):
self.db['quotes'].insert_one(dict(item))
return item
This example connects to a MongoDB instance and inserts each scraped item into a quotes collection.
Dealing with Anti-Scraping Mechanisms
As you begin scraping larger and more complex websites, you’ll likely encounter anti-scraping measures designed to block bots. Websites use various techniques like CAPTCHA, rate limiting, and IP blocking to prevent automated scraping. In this section, we’ll explore common anti-scraping mechanisms and how to overcome them using Scrapy.
Handling CAPTCHA
CAPTCHA (Completely Automated Public Turing test to tell Computers and Humans Apart) is one of the most common anti-bot techniques. Since CAPTCHA is designed to differentiate between bots and humans, it poses a significant challenge for web scrapers.
There are a few ways to handle CAPTCHA in Scrapy:
Manual CAPTCHA Solving: The simplest solution is to pause the scraping process when a CAPTCHA is encountered and solve it manually. However, this isn’t scalable for large-scale scraping.
Using Third-Party CAPTCHA Solvers: You can integrate third-party services like 2Captcha or Anti-Captcha to solve CAPTCHAs programmatically. These services require you to send the CAPTCHA image, and they return the solution.
Example using 2Captcha:
import requests
def solve_captcha(captcha_image_url):
api_key = 'your_2captcha_api_key'
response = requests.post(
'http://2captcha.com/in.php',
data={'key': api_key, 'method': 'base64', 'body': captcha_image_url}
)
captcha_id = response.text.split('|')[1]
captcha_solution = requests.get(f'http://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}')
return captcha_solution.text.split('|')[1]
Using Selenium with Scrapy: Some CAPTCHA types, like Google reCAPTCHA, require interaction with a browser. You can use Selenium to automate CAPTCHA-solving and pass the results back to Scrapy. Though this may work for simple visual CAPTCHAs, reCAPTCHA v3 and other complex CAPTCHAs may require a paid service or even more sophisticated approaches.
Bypassing IP Blocking and Rate Limiting
Websites often block IPs that send too many requests in a short period. Scrapy allows you to implement techniques that can avoid IP blocks:
Rotating Proxies: Using multiple proxy servers helps spread out your requests, preventing your IP from being blocked. You can integrate Scrapy with proxy services like ScraperAPI, Bright Data, or free proxy lists.
Example of proxy usage in Scrapy:
# In settings.py
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.httpproxy.HttpProxyMiddleware': 1,
}
# In your spider
def start_requests(self):
for url in self.start_urls:
yield scrapy.Request(url, callback=self.parse, meta={'proxy': 'http://your.proxy.url'})
Rotating User Agents: Websites often block bots based on their user agent string (which identifies the type of browser making the request). Scrapy can randomly rotate user agents to avoid detection.
You can add the scrapy-user-agents library to your project:
pip install scrapy-user-agents
Then configure your Scrapy settings:
# In settings.py
DOWNLOADER_MIDDLEWARES = {
'scrapy_user_agents.middlewares.RandomUserAgentMiddleware': 400,
}
Download Delays: To avoid being rate-limited or banned, you can introduce delays between requests.
In settings.py, set a random delay between requests:
DOWNLOAD_DELAY = 2 # 2 seconds between requests
RANDOMIZE_DOWNLOAD_DELAY = True
Using Scrapy Middleware to Avoid Detection
Scrapy provides middleware components that you can customize to handle specific aspects of the scraping process. You can create or use existing middleware for tasks like rotating proxies, spoofing headers, or managing cookies. These customizations help avoid detection by mimicking human browsing behavior.
Optimizing Scrapy for Performance
When scraping at scale, you need to optimize Scrapy for both speed and efficiency. Proper optimization ensures your spider can scrape large amounts of data quickly while minimizing the risk of being blocked.
Concurrent Requests and Threading
Scrapy allows you to control the number of concurrent requests made to a website. Increasing this number can significantly improve the speed of your spider, especially when scraping large sites.
In settings.py, configure the number of concurrent requests:
CONCURRENT_REQUESTS = 16
However, be cautious—sending too many requests simultaneously might get your IP blocked by the server. To prevent this, combine concurrent requests with rotating proxies and download delays.
Avoiding Memory Bloat
Large-scale scraping projects can consume a lot of memory if not managed correctly. Scrapy allows you to control memory usage with features like:
Item Pipelines: Ensure that items are processed and stored as they are scraped, reducing memory consumption. You can also write items to files (or databases) as soon as they are scraped.
Using Scrapy’s JOBDIR: Scrapy has a feature called JOBDIR that allows you to store the spider’s state in a directory, so if the spider is interrupted, it can resume from where it left off.
Example:
scrapy crawl myspider -s JOBDIR=crawls/jobdir
Scrapy Extensions and Caching
Scrapy provides caching capabilities via the HttpCacheMiddleware. This extension caches responses and reuses them on subsequent runs to avoid hitting the same pages multiple times, thus improving performance.
To enable caching, add the following to your settings.py:
HTTPCACHE_ENABLED = True
HTTPCACHE_EXPIRATION_SECS = 3600 # Cache expiration (1 hour)
HTTPCACHE_DIR = 'httpcache'
Conclusion and Best Practices
Web scraping with Scrapy is a powerful and scalable solution for extracting data from the web. By understanding the basics of spiders, using advanced techniques to handle dynamic content and anti-scraping mechanisms, and optimizing performance, you can build robust web scrapers that efficiently gather data for a wide range of applications.
Best Practices for Web Scraping:
Respect website rules: Always review a website’s robots.txt file and comply with their terms of service. Unethical scraping can lead to legal issues.
Use polite scraping techniques: Implement download delays, rotate user agents, and limit the frequency of requests to avoid overloading servers.
Test your spider: Run your spider on a few pages before scaling to ensure it behaves as expected and that data is being extracted correctly.
Handle errors gracefully: Websites can change without notice. Ensure your spider can handle unexpected issues like missing data or page errors.
By following these best practices and making the most of Scrapy’s features, you can create efficient, ethical, and scalable scraping solutions that gather valuable data for your projects.
That’s the full guide to Web Scraping with Scrapy in Python!
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends