10 Python Interview Questions You Should Know
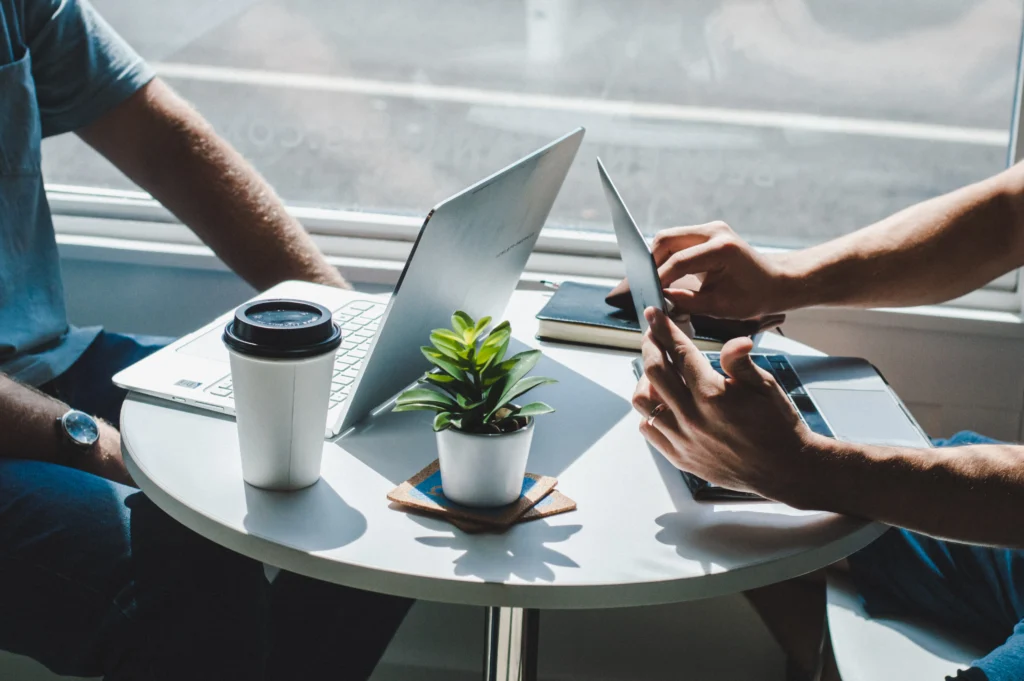
In the ever-evolving world of technology and programming, Python has emerged as a dominant force. Whether you are a seasoned developer or just starting your journey in the coding realm, mastering Python is a valuable asset. Python’s versatility and simplicity make it a popular choice for a wide range of applications, from web development to data analysis. If you are looking to land a Python-related job or advance your career, you might have to face a Python interview.
To help you prepare, this article explores ten essential Python interview questions and provides in-depth answers to each.
What is Python, and what are its key features?
Python is a high-level, interpreted programming language known for its simplicity and readability. Its key features include:
- Readability: Python uses a clean and easy-to-understand syntax, which reduces the cost of program maintenance.
- Versatility: Python can be used for web development, data analysis, artificial intelligence, and more.
- Interpretation: Python code is executed line by line, making it easy to debug.
- Community and Libraries: Python has a vast community and a rich ecosystem of libraries that extend its functionality.
Explain the differences between Python 2 and Python 3
Python 2 and Python 3 are two different versions of Python with some significant differences:
- Print Statement: In Python 2, you use `print` as a statement, while in Python 3, it’s a function, requiring parentheses.
- Unicode: Python 2 doesn’t fully support Unicode, whereas Python 3 handles it gracefully.
- Integer Division: Python 2 uses integer division by default, while Python 3 returns a float.
What is a Python variable, and how do you declare one?
A variable in Python is a name associated with a value. You can declare a variable simply by assigning a value to it, e.g., `x = 5`.
Python uses dynamic typing, which means a variable’s type is determined by its value.
Differentiate between lists and tuples in Python
Both lists and tuples are used to store collections of items, but the key difference is that lists are mutable (can be changed), while tuples are immutable (cannot be changed). To create a list, you use square brackets, like `my_list = [1, 2, 3]`, and for a tuple, you use parentheses, like `my_tuple = (1, 2, 3)`.
# Lists (mutable)
my_list = [1, 2, 3]
my_list.append(4) # Valid operation
my_list[0] = 10 # Valid operation
# Tuples (immutable)
my_tuple = (1, 2, 3)
# my_tuple.append(4) # Invalid operation - Tuples are immutable
# my_tuple[0] = 10 # Invalid operation - Tuples are immutable
Explain what is PEP 8 in Python
PEP 8, or Python Enhancement Proposal 8, is the style guide for writing clean and maintainable Python code. It covers topics like indentation, naming conventions, whitespace, and more. Adhering to PEP 8 guidelines is essential for writing Python code that’s easy to understand and collaborate on.
What are decorators in Python, and how do they work?
Decorators are a powerful feature in Python used to modify the behavior of functions or methods. They are often used for tasks like logging, authentication, and performance monitoring. A decorator is a function that takes another function as an argument and extends its behavior without modifying its code. Decorators are prefixed with the `@` symbol and placed above the function they decorate.
In Python, decorators are a powerful way to modify or enhance the behavior of functions or methods. Decorators are often used to add functionality to existing functions without modifying their source code.
Here’s a basic example of how decorators work:
# Define a simple decorator function
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
# Use the decorator with the "@" symbol
@my_decorator
def say_hello():
print("Hello!")
# Call the decorated function
say_hello()
When you call say_hello(), it produces the following output:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
Describe Python’s Global Interpreter Lock (GIL)
The Global Interpreter Lock (GIL) is a mutex in CPython (the standard Python implementation) that allows only one thread to execute in the interpreter at a time.
This means that in multi-threaded Python programs, only one thread can execute Python bytecode at a time. The GIL can limit the performance of multi-threaded Python applications, especially on multi-core processors.
What is the difference between a shallow copy and a deep copy in Python?
A shallow copy of an object is a copy of the object itself, but not its nested objects. In contrast, a deep copy creates a new object and recursively copies all the objects found within the original object.
The `copy` module in Python provides functions for making both shallow and deep copies of objects.
import copy
# Shallow Copy
original_list = [1, [2, 3], 4]
shallow_copied_list = copy.copy(original_list)
shallow_copied_list[1][0] = 20 # This change affects the original list as well
print("Original List:", original_list)
print("Shallow Copied List:", shallow_copied_list)
# Deep Copy
original_list = [1, [2, 3], 4]
deep_copied_list = copy.deepcopy(original_list)
deep_copied_list[1][0] = 20 # This change does not affect the original list
print("Original List:", original_list)
print("Deep Copied List:", deep_copied_list)
Output:
Original List: [1, [20, 3], 4]
Shallow Copied List: [1, [20, 3], 4]
Original List: [1, [2, 3], 4]
Deep Copied List: [1, [20, 3], 4]
What is the purpose of the `__init__` method in Python classes?
The `__init__` method is a special method in Python classes and serves as a constructor. It is automatically called when an instance of the class is created. You can use this method to initialize the attributes of the class, making it ready for use.
How can you handle exceptions in Python, and what is the purpose of the try, except, and finally blocks?
In Python, you can handle exceptions using the `try`, `except`, and `finally` blocks. The `try` block contains the code that might raise an exception. If an exception is raised, it is caught in the `except` block, where you can define how to handle the exception.
The `finally` block is optional and is executed regardless of whether an exception is raised or not. It is often used for cleanup operations.
try:
# Code that may raise an exception
x = 10 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
# Code to handle the specific exception
print("Division by zero is not allowed.")
except Exception as e:
# Code to handle other exceptions
print(f"An error occurred: {e}")
finally:
# Code that will always run, with or without an exception
print("Finally block always executed")
print("After the try-except-finally block")
These ten Python interview questions and their detailed answers should help you in your quest to master Python and excel in your interviews. Remember to not only memorize the answers but also understand the underlying concepts to showcase your proficiency as a Python developer.
Good luck with your Python interview!
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends