Django Tutorial for Beginners – Part 2
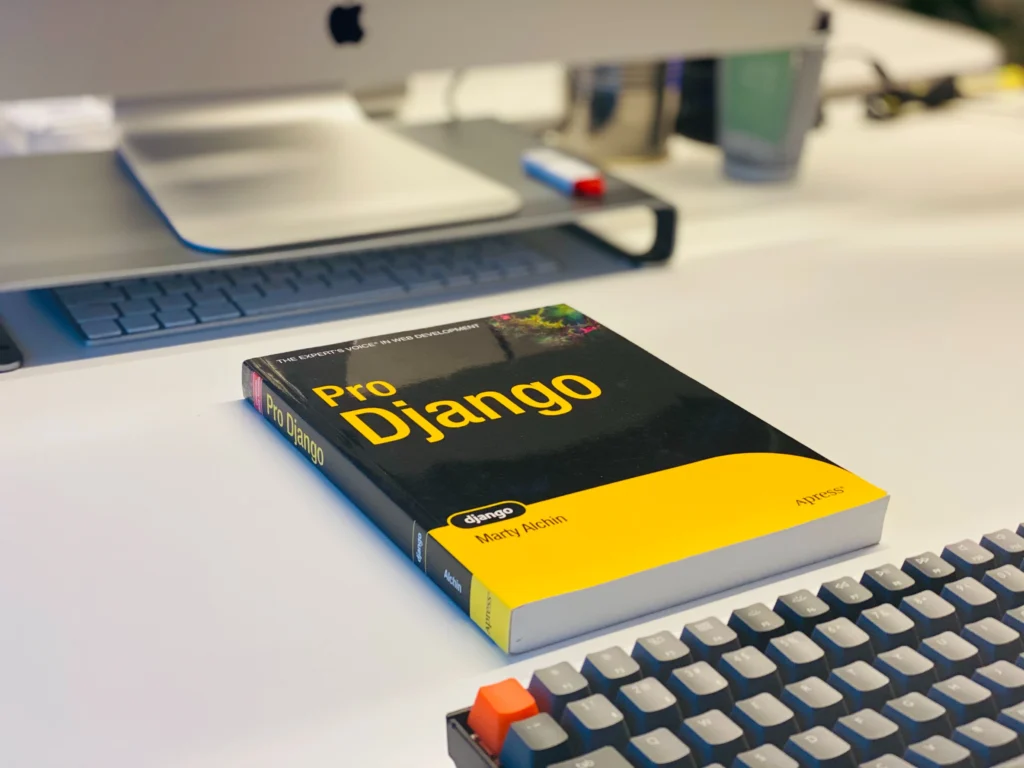
If you’re a web developer or an aspiring one, you’ve probably heard of Django. It’s a powerful and versatile Python web framework that makes building web applications a breeze. In the world of Django, three key concepts play a vital role in creating dynamic and functional websites: URLs, views, and models.
In this comprehensive guide, we’ll explore these fundamental elements of Django, understand their roles, and learn how to use them effectively to develop web applications that stand out.
Here is Part 1 of this Django Tutorial: Django Tutorial Part 1.
Before we dive into the nitty-gritty of URLs, views, and models in this Django tutorial, let’s first establish a clear understanding of what these terms mean before jumping.
What Are URLs, Views, and Models?
URLS
In the context of Django, URLs are used to define the structure of your website’s navigation. They determine how the different parts of your web application are accessed through a web browser. URLs act as a map to direct users to the appropriate views, allowing them to interact with your website’s content.
Views
Views in Django are responsible for processing the incoming web requests, performing any necessary logic, and returning an HTTP response. Think of views as the bridge between your website’s URLs and its underlying data. They define what the user sees and how they can interact with it.
Models
Models are a fundamental component of Django’s Object-Relational Mapping (ORM) system. They define the structure of your application’s database tables, specifying the fields and relationships between data entities. Models make it easy to work with your database, allowing you to perform operations like creating, retrieving, updating, and deleting records.
Now that we have a solid foundation, let’s delve deeper into each of these components.
Working with URLs
Defining URLs in Django
In Django, URL patterns are defined in your application’s “urls.py” file. You specify the URL patterns and associate them with views, effectively mapping the URLs to the appropriate code that should be executed when a user accesses a particular URL.
Here’s a simple example of defining a URL pattern in Django’s “urls.py”:
from django.urls import path
from . import views
urlpatterns = [
path('home/', views.home, name='home'),
]
In this example, when a user navigates to the ‘/home/’ URL, the “home” view function is executed.
Using Regular Expressions
Django also provides support for using regular expressions in URL patterns. This flexibility allows you to create dynamic URLs and capture variables from the URL to be used in your views. For example:
from django.urls import path
from . import views
urlpatterns = [
path('articles/<int:article_id>/', views.article_detail, name='article_detail'),
]
In this case, the “<int:article_id>” part captures an integer from the URL and passes it to the “article_detail” view function.
Creating Views
Defining Views in Django
Views in Django are Python functions that take a web request and return a web response. They encapsulate the logic for processing requests, interacting with the database, and rendering templates.
from django.http import HttpResponse
def home(request):
return HttpResponse("Welcome to our website!")
Here’s a simple example of a Django view:
In this example, the “home” view simply returns a “Welcome to our website!” message when the ‘/home/’ URL is accessed.
You might like: 5 Advanced concepts in Python to become a pro
Class-Based Views
While the function-based views are straightforward, Django also offers class-based views, which provide a more organized way to handle complex views. Class-based views are highly reusable and offer various built-in mixins for common tasks, such as authentication and form handling.
An example of a class-based view is given below:
# views.py
from django.shortcuts import render
from django.views import View
from .models import Item
class ItemListView(View):
template_name = 'item_list.html'
def get(self, request):
items = Item.objects.all()
return render(request, self.template_name, {'items': items})
Working with Models
Defining Models in Django
Models in Django define the structure of your database tables. You define models as Python classes, specifying the fields and relationships between them. Django then automatically generates the necessary SQL to create the corresponding database tables.
Here’s an example of defining a simple model in Django:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
price = models.DecimalField(max_digits=10, decimal_places=2)
In this case, we’ve defined a “Product” model with fields for the product’s name, description, and price.
Creating Database Tables
Once you’ve defined your models, you need to create the corresponding database tables. Django provides a convenient management command for this:
python manage.py makemigrations
python manage.py migrate
These commands generate migration files and apply the changes to the database, creating the necessary tables.
Relationships between URLs, Views, and Models
The magic of Django happens when you bring URLs, views, and models together. Let’s explore how these components interact.
Linking URLs to Views
In your “urls.py”, you define URL patterns and associate them with view functions or class-based views. When a user accesses a URL, Django’s URL dispatcher routes the request to the appropriate view, passing any captured URL variables for processing.
Interacting with Models in Views
Views often need to interact with models to retrieve or manipulate data. Django provides an ORM that allows you to work with models in a Pythonic way. You can query the database, create new records, update existing ones, and delete data, all from your view functions.
Here’s an example of a view that retrieves a list of products from the “Product” model and displays them:
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
return render(request, 'products/product_list.html', {'products': products})
In this example, the view retrieves all products from the “Product” model and renders them using a template.
Conclusion
URLs, views, and models are the backbone of any Django web application. Understanding how these components work together is crucial for building dynamic and functional websites. Now, armed with this knowledge, you’re well on your way to creating powerful, SEO-friendly web applications using this Django Tutorial.
Happy coding!
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends