For Loops in Python, A complete guide
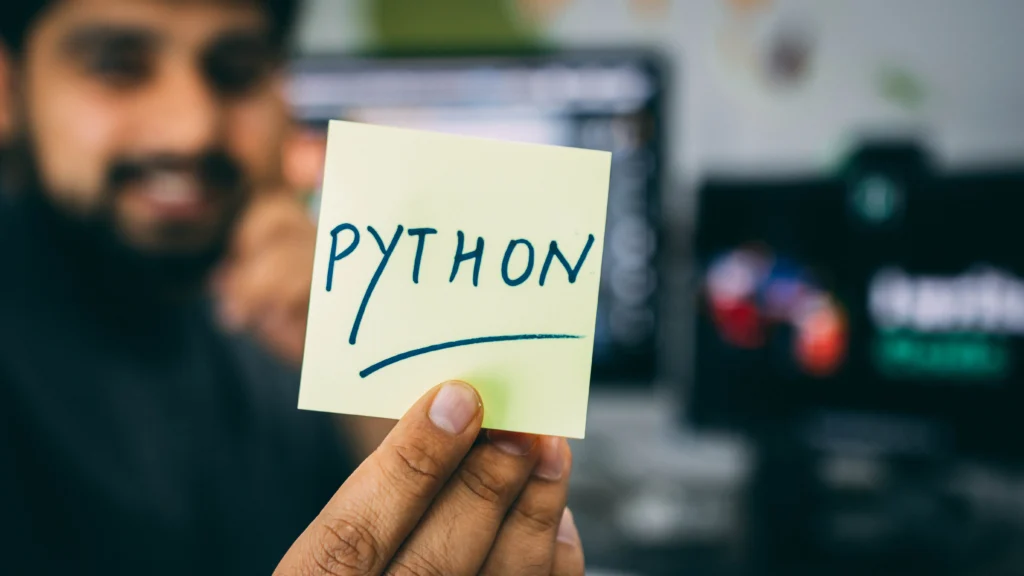
For loops are widely used in Python Programming language. By using For loops in Python, we are asking the IDE to loop over that statement(s) multiple times until the termination condition triggers.
Loops are used in Python for iterating over an iterable sequence.
So, let’s start and learn Python for loops
A simple For Loop
laptops = ['Hp', 'Lenovo', 'Dell']
for laptop in laptops:
print(laptop)
OUTPUT:
Hp
Lenovo
Dell
In this For loop, we created a simple for loop which iterates over a list. This list is an iterable data structure consisting of multiple Laptop names. It prints the laptop name and loops over it till it reaches the termination condition.
The termination condition is triggered after the last element is printed. In these simple loops, Python automatically calculates the length and stops at length – 1.
For loop with if else Statement
Let’s see how can we use a Python For Loop with an if statement:
nums = [1,2,3,4,5,6,7,8,9]
even = []
odd = []
for num in nums:
if num%2 == 0:
even.append(num)
else:
odd.append(num)
print(even)
print(odd)
OUTPUT:
[2, 4, 6, 8]
[1, 3, 5, 7, 9]
Here, we have a list of numbers in the range of 1 to 9. We are using an if statement to check if the number is even by checking if it is divisible by 2. If it is then we append/add it to our list named even. Otherwise, we add it to the list named odd as it is not divisible by 2.
Continue Statement in For loops in Python
Now, let’s see the use of the continue statement in For Loops:
laptops = ['Hp', 'Lenovo', 'Dell']
for laptop in laptops:
if laptop == 'Hp':
continue
print(laptop)
OUTPUT:
Lenovo
Dell
Here, we want to print all laptop names except the laptop named ‘HP’. Therefore, we use an if statement to check whether the target laptop name is found or not. If it is found, we ask the loop to continue and print the rest of the laptops.
For Loop with range
The range() method is used when a programmer wants to limit the number of iterations till a specific point.
Let’s consider this example:
for num in range(1, 6):
print(num)
OUTPUT:
1
2
3
4
5
In this Python loop, we are printing numbers from 1 to 6 using range().
Now, Let’s move on to some advanced ways how we can use Python for loops.
Nested For Loops in Python
Here’s an example of a nested for loop in Python:
laptops = ["Dell", "HP", "Lenovo"]
specs = ["i5", "i7", "AMD"]
# Nested loop to iterate over laptops and specs
for laptop in laptops:
for spec in specs:
print(laptop, spec)
OUTPUT:
Dell i5
Dell i7
Dell AMD
HP i5
HP i7
HP AMD
Lenovo i5
Lenovo i7
Lenovo AMD
As you can see, we have 2 lists i.e. Laptops and specs. In this nested for loop, we are making every possible combination between the two lists as shown in the output.
For loop in Python using enumerate()
There are scenarios where we need the index and its value to start solving the problem at hand. We can easily solve this problem using enumerate() method.
fruits = ["apple", "banana", "cherry", "durian"]
for index, fruit in enumerate(fruits):
print(index, fruit)
OUTPUT:
0 apple
1 banana
2 cherry
3 durian
As you can see, we have a list named fruits. When a list is enumerated, it returns an index, value pair.
For loops with tqdm
What if we want to see the loading bar and some stats while the for loop is in running, like iterations/second?
You can install tqdm by using this command
pip install tqdm
Let’s consider the below-mentioned example
from tqdm import tqdm
import time
for _ in tqdm(range(100)):
time.sleep(2)
OUTPUT:

In this scenario, we can use tqdm, which is a third-party library that allows for loops to show a status bar along with some stats of the loop. This is generally helpful when we have a lot of data and the loops take quite some time to complete. By using tqdm, we are aware of the progress status and the current number of iterations the loop is in.
You can explore more, you can check out the official documentation here.
If you want to learn more about Python, start by learning these top 5 advanced concepts.
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends