Tips for better Pythonic code
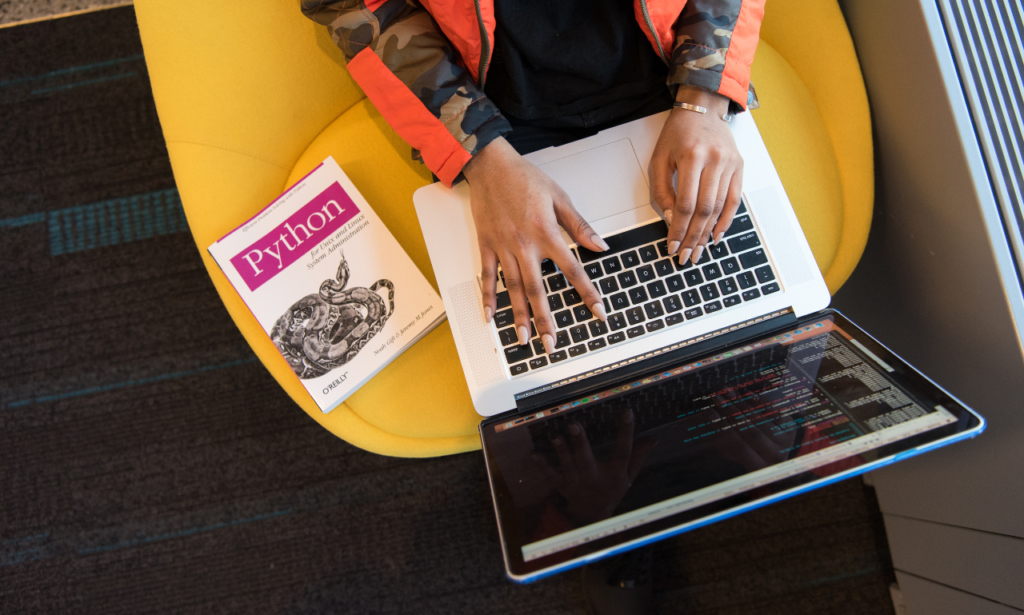
In this article, we will be talking about how to code better in Python.
So, starting off let’s see what Python is.
“ Python is a high-level, general-purpose programming language. Its design philosophy emphasizes code readability with the use of significant indentation via the off-side rule. Python is dynamically typed and garbage-collected “
As per StackOverflow’s analysis, Python is one of the hottest and most widely used programming languages around the globe in 2023. Our rankings show the same trend.
Getting started with Python is not that hard. You can learn the syntax and basics relatively quickly. But, there are certain rules that you should follow in order to write better Pythonic code.
Use annotations
Readability is very important while programming. The rule of thumb is that when someone else reads your code, it should be readable and the intent should be clear.
You can use annotations in parameters and methods too. These annotations do not bind the code to follow these conventions. These just simply show what the data types should be.
For example:
Compare these 2 methods:
#Method 1:
def add(a,b):
return a + b
#Method 2:
def add(a: int,b: int) -> int:
return a + b
Both methods take in arguments and return the sum of the passed arguments. The first method does not tell what the expected datatype of the passed argument is. While the 2nd method tells the reader the expected data types of parameters as well as the returned data type of this method.
This Python code (Method 2) will not run into any error if a float is passed. It will simply return the appropriate answer. So, python never enforces the data types, rather it is a convention for better readability.
Write the purpose of the method
While writing methods, you can write the purpose of the Python function/method.
You have 2 options:
1. Block Comments
In block comments, you can write the purpose of the method right after def with # followed by a single space.
For instance:
def add(a , b):
# returns the sum of two numbers
return a + b
2. Docstrings
Docstrings are another convention for documentation of your public methods. While it is not necessary for non-public methods, you can still use block comments for describing that method.
A docstring can be used like this:
def add(a , b):
""" returns the sum of two integers"""
return a + b
You can write about parameters like this too if you want to provide more details:
def add(a, b):
"""
returns the sum of two integers
:param a: about param a
:param b: about param b
:return: what are we returning and why
"""
return a + b
Follow conventions for naming variables, methods, and classes:
Every programming language has its naming convention. In Python, the naming convention for different entities is as follows:
Class → we follow camel notation i.e. Class, Recommend, RecommendItems
Class → we follow camel notation i.e. Class, Recommend, RecommendItems
Variables → variable, no_of_items
Constants → CONSTANTS, ITEMS, ITEMS_TO_RECOMMEND
Line Length and Code Length
The character length on a single line is 72 – 99. This rule applies to docstrings and codes both. Again, these rules ensure the readability of code. Code readability goes a long way when we are working in teams.
Use list comprehensions
In Python, loops are quite common and are used widely. In order to work with tables like lists, dict, tuples, etc., loops are the number one choice.
For loops can slow down the code, mainly because it is spanned over certain lines (even for the most basic ones). The interpreter has to read and loop through the lines multiple times till the loop terminates. But, when the for loops begin to get complex, it is recommended to use conventional for loops.
For instance, we need to write a for loop which returns a list with 0 at even numbers and 1 at odd numbers.
Input → [1,2, 3, 4, 5, 6, 7, 8, 9, 10]
Output → [1, 0, 1, 0, 1, 0, 1, 0, 1, 0]
A simple for loop would do the trick:
Now, the code is:
- In fewer lines
- Provides Faster execution
- Easier to understand
If you want to dig deeper and learn more about best practices for Python, you can refer to pep8. It is highly recommended for ensuring readability and consistency in Python programming.
Talha is a seasoned Software Engineer with a passion for exploring the ever-evolving world of technology. With a strong foundation in Python and expertise in web development, web scraping, and machine learning, he loves to unravel the intricacies of the digital landscape. Talha loves to write content on this platform for sharing insights, tutorials, and updates on coding, development, and the latest tech trends